Exceeding Local Storage Capacity
Introduction
Have you ever considered the capacity when using LocalStorage in your project?
Depending on the purpose of localStorage, it might not be something you often think about. However, if you're storing data that accumulates over time, the data can exceed the localStorage capacity.
What happens when it exceeds? 초과하면 과연 어떻게 동작할까요?
The answer is It throws an error.
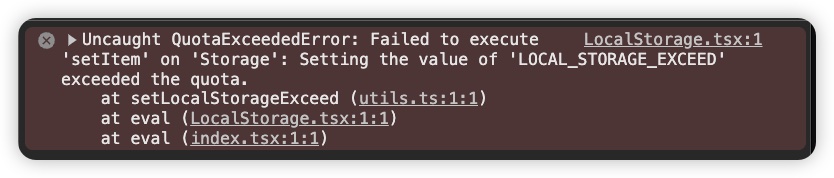
Solution
When the error occurs, the setStorage
statement terminates due to the throw, and the error propagation begins.
If your project handles error propagation well, the error message will be logged, and if not, the project might stop.
To prevent this situation, you should use a try-catch
block to catch the error and add logic to handle it.
const setStorage = (key, value) => {
try {
window.localStorage.setItem(key, value);
} catch (e) {
// Stop Throw Error
console.error('Local Storage Exceed Error', e);
}
}
Of course, an error indicates that the localStorage capacity has been exceeded, so the user will continue to encounter Local Storage Exceed Errors and won't be able to save data.
Therefore, in addition to error handling, you should also include logic to clear the data.
const setStorage = (key, value) => {
try {
window.localStorage.setItem(key, value);
} catch (e) {
// Stop Throw Error
console.error('Local Storage Exceed Error', e);
window.localStorage.clear();
}
}
Try it yourself
Now that you know localStorage has a capacity limit and how to handle errors, if you're curious, you can try filling up the localStorage yourself.
Click the Play icon below to start filling localStorage with data. While it depends on the browser, it generally has a capacity of about 5MB.
Click Play! It won't take more than a minute.
Fill `LOCAL_STORAGE_EXCEED` Storage
When you see the Local Storage Exceed Error in the gray console, it means the localStorage is full. You can also check the error object in the actual browser console by opening the developer tools.
What if you use a different key? Does it have a different capacity per key?
Fill `ANOTHER_STORAGE_KEY` Storage
The result, unsurprisingly, is the same. The capacity of localStorage is managed per browser, so it remains the same.
Even if you open a new tab in the same browser, the storage remains full, as it is shared across tabs.
Conclusion
- LocalStorage has a capacity of about 5MB in Chrome.
- Exceeding the localStorage capacity triggers a Local Storage Exceed Error.
- You should add logic to catch and handle the error.