Local Storage 용량 초과
Introduction
프로젝트에서 LocalStorage를 사용했을 때 용량을 고려한 적이 있나요?
localStorage의 용도에 따라 다르겠지만, 대부분의 경우는 용량을 고려하지 않고 사용하게 됩니다. 하지만, 데이터의 성격이 계속해서 쌓이는 구조의 데이터를 저장한다면 데이터는 localStorage의 용량을 초과할 수 있습니다.
초과하면 과연 어떻게 동작할까요?
정답은 Throw 에러 입니다.
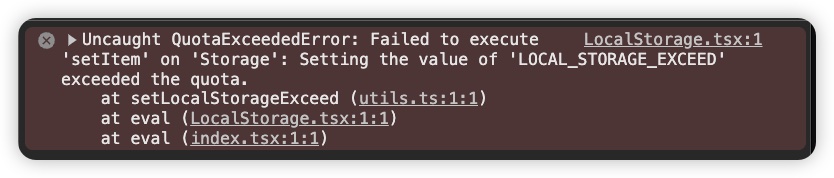
Solution
위 에러가 발생하면, setStorage
구문은 throw로 인해 종료가 되고 에러 전파를 진행하게 됩니다.
프로젝트에 에러 전파를 잘 처리하고 있다면, 에러 메세지와 함께 에러 로그가 수집 될 것이고 전파를 잘 처리 하지 않았다면 프로젝트가 멈출 수 있습니다.
이러한 상황을 방지하기 위해서는 try-catch
구문을 사용해서 에러를 잡아내고, 에러를 처리하는 로직을 추가해야 합니다.
const setStorage = (key, value) => {
try {
window.localStorage.setItem(key, value);
} catch (e) {
// Stop Throw Error
console.error('Local Storage Exceed Error', e);
}
}
물론 에러가 발생했다는 것은 LocalStorage의 용량을 초과했다는 것이므로 앞으로 사용자는 계속해서 Local Storage Exceed Error가 발생하고, 데이터를 저장하지 못할 것 입니다.
따라서 에러처리 뿐 아니라 데이터를 지워주는 작업까지 추가해주어야합니다.
const setStorage = (key, value) => {
try {
window.localStorage.setItem(key, value);
} catch (e) {
// Stop Throw Error
console.error('Local Storage Exceed Error', e);
window.localStorage.clear();
}
}